There are lots of modules available for
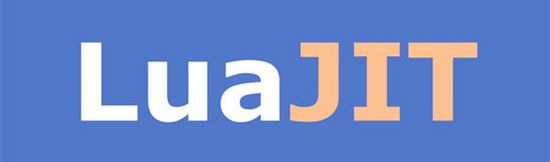
Contents
Embedding regular versions of Lua
See here for information on how to compile
Lua interpreter
In Nift's interpreter mode the prompt will just tell you which language you are using. If you would like the prompt to also display the present working directory (up to using half the width of the console) you can switch to the shell mode using
You can switch to one of the other languages available in Nift's interpreter using
Running Lua scripts
If you have a
nsm run path/script-name.lua nift run path/script-name.lua
If the script has a different extension, say
nsm run -lua path/script-name.ext nift run -lua path/script-name.ext
ExprTk from Lua
You can run
exprtk("ExprTk code")
Note: When using the interpreter or running a script the ability to call
Lua from f++
You can run
lua(--[[ line of Lua code ]]--)
lua { -- block of Lua code }
Lua from N++
You can run
@lua(--[[ line of Lua code ]]--)
@lua { -- block of Lua code }
Nift functions
The following functions specific to Nift may be made available inside
syntax | example | about |
---|---|---|
change directory | ||
compile and evaluate |
||
compile |
||
compile |
||
evaluate |
||
evaluate |
||
load |
||
return string of compiled |
||
return string of compiled |
||
set Nift variable from number | ||
set Nift variable from string | ||
get pointer to Nift variable | ||
get number from Nift variable | ||
get string from Nift variable | ||
write to console, output file (ofile), or a stream | ||
execute system call/command | ||
syntax | example | about |
An example of using
string str="hello, world!" lua_addnsmfns() lua { nsm_write(console, nsm_tostring("str"), endl) nsm_setstring("str", "hello, mars!") nsm_write(console, nsm_tostring("str"), endl) }
LuaRocks
As mentioned above, LuaRocks may be used to install all sorts of modules for Lua. There is information below about installing LuaRocks on different platforms and configuring your platform.
Note: Some rocks are to interface with other programs that you also need to have installed on your machine, for example LuaSQL-MySQL expects you to have
Some of the rocks you might find useful with Nift are:
- cloud_storage - access Google Cloud Storage from Lua
- Lua-cURL - binding to libcurl (official website, GitHub)
- lua-discount - binding to a fast C implementation of the Markdown text-to-html markup system (official website)
- etlua - allows you to render ERB style templates but with Lua. Supports <% %>, <%=%> and <%- %> tags (with optional newline slurping) for embedding code
- lua-cjson - fast JSON encoding/parsing support
- luajwt - JSON Web Tokens for Lua, very fast and compatible with pyjwt, php-jwt and ruby-jwt
- graphql - Lua GraphQL implementation (GitHub)
- luahaml - implementation of the Haml markup language for Lua
- http - HTTP library for Lua: optionally asynchronous (including DNS lookups and TLS), supports HTTP(S) version 1.0, 1.1 and 2, functionality for both client and server, cookie Management, websockets
- lustache - allows you to use the Mustache templating standard in Lua by passing in a string, data, and partial templates. It precompiles and caches templates for speed, and allows you to build complex strings such as html pages by iterating through a table and inserting values (official website)
- mailgun - send email with Mailgun
- Markdown - pure-lua implementation of the Markdown text-to-html markup system
- magick - bindings to ImageMagick for LuaJIT using FFI
- moonscript - programmer friendly language that compiles to Lua (official website)
- LuaSQL-MySQL - database connectivity for Lua (MySQL driver), enables a Lua program to connect to databases, execute arbitrary SQL statements and retrieve results in a row-by-row cursor fashion
- lsqlite3 - a binding for Lua to the SQLite3 database library
- LuaSQL-SQLite3 - database connectivity for Lua (SQLite3 driver), enables a Lua program to connect to databases, execute arbitrary SQL statements and retrieve results in a row-by-row cursor fashion
- nixio - a multi-platform library offering a wide variety of features such as IPv4, IPv6 and UNIX networking, large file I/O, file system operations, system and process control, POSIX user/group management, basic cryptographical hashing, hmac and TLS support, bit operations and binary conversion (also see here)
- pgmoon - PostgreSQL driver written in pure Lua for use with OpenResty's cosocket API. Can also be used in regular Lua with LuaSocket and LuaCrypto
- redux-lua - implements redux using Lua language
- lua-requests - HTTP requests made easy! Support for Basic Auth, Digest Auth. HTTP response parsing has never been easier (GitHub)
- lua-resty-template - templating engine (HTML) for Lua and OpenResty
- LuaSocket - an extension library that is composed by two parts: a C core that provides support for the TCP and UDP transport layers, and a set of Lua modules that add support for functionality commonly needed by applications that deal with the Internet
- web_sanitize - library for sanitizing untrusted HTML
- lua-vips - a binding for the libvips image processing library. it is usually faster and needs less memory than similar libraries
- LuaFileSystem - offers a portable way to access the underlying directory structure and file attributes
- lua-path - file system path manipulation library
- lanes - multithreading support for Lua
- lua-llthreads2 - low-level threads for Lua, in additional module supports: thread join with zero timeout; logging thread errors with custom logger; run detached joinable threads; pass cfunctions as argument to child thread
- luasystem - platform independent system calls
- sys - provides system functionalities for Torch
- LPeg - new pattern-matching library for Lua, based on Parsing Expression Grammars (PEGs)
- luaossl - most comprehensive OpenSSL module in the Luaverse
- LuaCrypto - frontend to the OpenSSL cryptographic library, the OpenSSL features that are currently exposed are: digests (MD5, SHA-1, HMAC, and more), encryption, decryption and crypto-grade random number generators
- MD5 - offers basic cryptographic facilities: a hash (digest) function, a pair crypt/decrypt based on MD5 and CFB, and a pair crypt/decrypt based on DES with 56-bit keys
- luaposix - library binding various POSIX APIs (see also lunix)
- xml - fast xml parser based on RapidXML
- lyaml - libYAML binding for Lua, read and write YAML format files with Lua
- yaml - Lua YAML serialization using LibYAML, LibYAML is generally considered to be the best C YAML 1.1 implementation
Other Lua resources worth checking out include:
- Fengari - the Lua VM written in JavaScript, it uses JavaScript's garbage collector so that interoperability with the DOM is non-leaky (GitHub)
- FFI Library - allows calling external C functions and using C data structures from pure Lua code
- libgit2 - portable, pure C implementation of the Git core methods provided as a re-entrant linkable library with a solid API, allowing you to write native speed custom Git applications in any language which supports C bindings (luagit2 - Lua bindings for libgit2)
- SciLua - Scientific computing with LuaJIT
- Torch - a scientific computing framework with wide support for machine learning algorithms that puts GPUs first
You can also compile the following languages to Lua (see here for a longer list):
- Amulet - simple, functional programming language in the ML tradition
- CSharp.lua - C# to Lua compiler
- Fennel - a programming language that brings together the speed, simplicity, and reach of Lua with the flexibility of a lisp syntax and macro system
- Haxe - an open source high-level strictly-typed programming language with a fast optimizing cross-compiler
- MoonScript - a dynamic scripting language that compiles into Lua
- TypeScriptToLua - generic TypeScript to Lua transpiler. Write your code in TypeScript and publish Lua!
- Urn - a Lisp dialect with a focus on minimalism
Installing LuaRocks on FreeBSD
Instructions for installing
sudo pkg install devel/lua-luarocks
If you installed
sudo micro /usr/local/etc/luarocks/config-5.2.lua
Then modify the appropriate lines so that the configuration file has the following:
lua_interpreter = "lua5.1"; lua_version = "5.1"; variables = { LUA_DIR = "/usr/local"; LUA_INCDIR = "/usr/local/include/lua51"; LUA_BINDIR = "/usr/local/bin"; }
Once
You should then be able to install rocks using for example:
sudo luarocks install LuaFileSystem
Installing LuaRocks on Linux
sudo apt-get install luarocks
You should then be able to install rocks using for example:
sudo luarocks install LuaFileSystem
Once
Installing LuaRocks on OSX
The easiest way to install LuaRocks on OSX is to use homebrew with:
brew install luarocks
Note however that this typically sets
luarocks install moonscript --lua-version=5.1
To change the
- run
luarocks and note where the system configuration file is located; - run
sudo nano system-config-path and make sure it has the following two lines:
lua_interpreter = "lua5.1" lua_version = "5.1"
Once
Note: If you do not configure
Alternatively, assuming you have command line tools installed for XCode, you can download the latest
./configure --lua-version=5.1 make sudo make install
Then you can install rocks using for example:
sudo luarocks install lua-cjson
Same as above, once
Installing LuaRocks on Windows
LuaRocks can be installed through Chocolatey with:
choco install luarocks
You can supposedly also install
INSTALL.BAT /MW /L
You should then be able to install rocks using for example:
sudo luarocks install LuaFileSystem
Once
Moonscript functions
Suppose you have a moonscript file
func1 = -> print "hello, world!" func2 = -> print "hello, mars!" { :func } { :func2 }
Inside
require "moonscript" fns = require "functions" fns.func() fns.func2()